ConsciousnessAPI Quickstart
Getting Started
Before you begin, you'll need an API key to authenticate your requests. To get your API key:
- Sign up for an account if you haven't already
- Visit your account page
- Click "Create API Key" and give your key a label
- Copy your API key and store it securely - you won't be able to see it again!
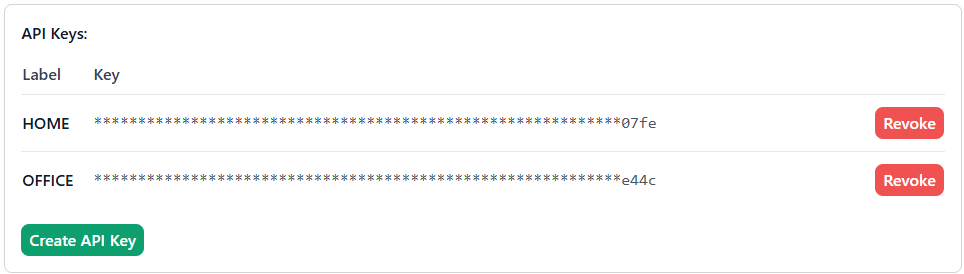
Installation
Install our Python library using pip to easily interact with the ConsciousnessAPI. This package provides a simple interface to access all API endpoints.
pip install https://consciousnessproject.xyz/consciousness_api-0.1.1.tar.gz
Managing Your API Key
There are several secure ways to manage your API key:
Environment Variable
Set your API key as an environment variable (recommended for production):
# Linux/Mac
export CONSCIOUSNESS_API_KEY='your-api-key'
# Windows PowerShell
$env:CONSCIOUSNESS_API_KEY='your-api-key'
Using a .env File
Create a .env
file in your project root (remember to add it to .gitignore
):
# .env
CONSCIOUSNESS_API_KEY=your-api-key
Then in your code:
from dotenv import load_dotenv
import os
load_dotenv() # Load environment variables from .env file
api_key = os.getenv("CONSCIOUSNESS_API_KEY")
Interactive Input
For development or scripts, you can prompt for the API key securely:
import os
from getpass import getpass
from consciousness_api import ConsciousnessAPI
# Try to get API key from environment first
api_key = os.getenv("CONSCIOUSNESS_API_KEY")
# If not found, prompt securely
if not api_key:
api_key = getpass("Enter your API key: ")
api = ConsciousnessAPI(api_key=api_key)
Basic Usage
import os
from consciousness_api import ConsciousnessAPI
# Set your API key using an environment variable
api_key = os.getenv("CONSCIOUSNESS_API_KEY")
# Initialize the client
api = ConsciousnessAPI(api_key=api_key)
# List all authors
authors = api.list_authors()
# Get an author
author = api.get_author(42)
# Create a theory
theory = api.create_theory(
name="Consciousness Theory",
description="A new theory about consciousness",
author_ids=[42]
)
For a complete API reference, visit the API Docs.